概要
Learn variables and operations in PHP while actually coding them.
準備
After installing PHP, let’s actually code it.
Change permissions
PHP’s “DocumentRoot” is “/ var / www / html” by default.
Since the html authority is the administrator, change it to general.
Change the owner with the chown command.
sudo chown username: username / var / www / html
index.php creation
Create an index.php file under the html folder.
vim /var/www/html/index.php
You can use vi instead of the vim editor.You can also create it with an editor and upload it.
PHP variables
Those who are new to programming may be confused.
Think of a variable as a “box” that stores “some data”.
For example, at a pizza shop, we will deliver “pizza” in a “box”. At home, open the “box” and take out the “pizza” to eat.
Of course, in addition to “pizza”, you can pack various things such as “potato” and “juice”.
constant
Some variables are “constants” that do not change their contents.
Once declared, the contents do not change during the processing of the program.
define("constant", "abc");
Declare a constant with define. The character string “abc” is stored in the constant name “constant”.
Variable.logical value
A logical value represents true or false.I’m not sure at first, but it is used for comparison results.
$temp = true;
Set true to the variable name “temp”.
Variable.integer
Set an integer value for the variable.
$temp = 123;
Set 123 to the variable name “temp”.
Variable. Floating point
Set the variable to a floating point number.
$temp = 123.456;
Set 123.456 to the variable name “temp”.
Variable.string
Set the character string to the variable.
$temp = "CODESE";
Set CODESE to the variable name “temp”.
It’s very easy. Depending on the language, it may be necessary to change the declaration according to the data or secure an area. You don’t need PHP. If you store more data than the area or forget to release it, serious things will happen.
PHP arithmetic
The four arithmetic operations are calculated using the symbols “+”, “-“, “/”, “*”.
Addition
$temp = 1 + 1;
Subtraction
$temp = 1 - 1;
Multiply
$temp = 2 * 2;
division
$temp = 2 / 2;
Operations using variables
Calculate using variables.
$temp10 = 10;
$temp20 = 20;
Addition
$temp = $temp10 + $temp20;
Subtraction
$temp = $temp10 - $temp20;
Multiply
$temp = $temp10 * $temp20;
division
$temp = $temp10 / $temp20;
Practice
Enter the code below to get it working.
All you have to do is access the IP address of the guest OS with a web browser.
For example, if “192.168.0.100” is the IP address of the guest OS, it will be as follows.
http://192.168.0.100/
<html>
<head>
<title>PHP</title>
</head>
<body>
<?php
// コメント
/* コメント */
/* 出力 */
// echo phpinfo();
echo '<p>Hello World</p>';
echo '<H1>Hello World<H1>';
echo '<H2>Hello World<H2>';
echo '<H3>Hello World<H3>';
/* 定数 */
define("constant", "abc");
print(constant); // 定数の中身"abc"が表示されます
print("<br/>"); // 改行
/* 変数.論理値 */
$temp = true; // boolean
print($temp); // "1"が表示されます
print("<br/>"); // 改行
/* 変数.整数 */
$temp = 123; // integer
print($temp); // "123"が表示されます
print("<br/>"); // 改行
/* 変数.浮動小数点 */
$temp = 123.456; // float
print($temp); // "123.456"が表示されます
print("<br/>"); // 改行
/* 変数.文字列 */
$temp = "CODESE"; // string
print($temp); // "CODESE"が表示されます
print("<br/>"); // 改行
/* 四則演算 */
$temp = 1 + 1; // 加算
print($temp); // "2"が表示されます
print("<br/>"); // 改行
$temp = 1 - 1; // 減算
print($temp); // "0"が表示されます
print("<br/>"); // 改行
$temp = 2 * 2; // 乗算
print($temp); // "4"が表示されます
print("<br/>"); // 改行
$temp = 2 / 2; // 除算
print($temp); // "1"が表示されます
print("<br/>"); // 改行
/* 四則演算.変数 */
$temp10 = 10;
$temp20 = 20;
$temp = $temp10 + $temp20; // 加算
print($temp); // "30"が表示されます
print("<br/>"); // 改行
$temp = $temp10 - $temp20; // 減算
print($temp); // "-10"が表示されます
print("<br/>"); // 改行
$temp = $temp10 * $temp20; // 乗算
print($temp); // "200"が表示されます
print("<br/>"); // 改行
$temp = $temp10 / $temp20; // 除算
print($temp); // "0.5"が表示されます
print("<br/>"); // 改行
?>
</body>
</html>
Execution result
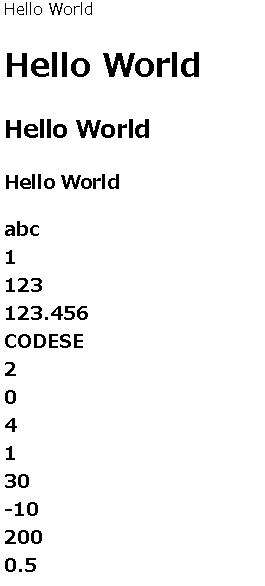
good job for today.